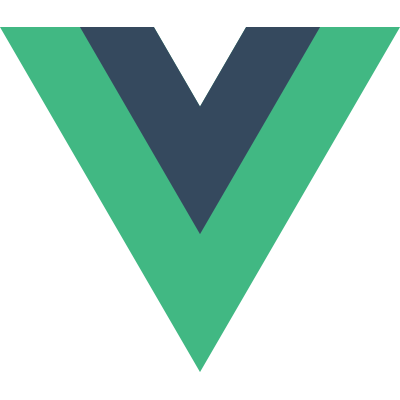
vue-service-model
Vue.js Library for handling REST service requests with caching, aggregation and model definitions.
Model definitions
Define your backend REST services as models and use them for simple usage in your frontend.
Service requests
Reduce your service request load with request caching and aggregation.
Field rendering
Define the display and input rendering of your fields at one point and receive consistent representation of your fields.
(opens new window)
(opens new window)
(opens new window)
(opens new window)
(opens new window)
# Installation
npm install vue-service-model
# Examples
Definition of a simple ServiceModel
without using fields. https://jsonplaceholder.typicode.com/albums/ is being used as an example REST JSON service.
import {ServiceModel, Field} from 'vue-service-model'
class Album extends ServiceModel {
// Define service url
static urls = 'https://jsonplaceholder.typicode.com/albums/'
// Define model fields
static fieldsDef = {
id: new Field({primaryKey: true}),
title: new Field()
}
}
// Retrieve all albums from /albums/
const allAlbums = await Album.objects.list()
// Retrieve specific album from /albums/1/
const album = await Album.objects.detail(1)
// Retrieve value for field title
await album.val.title // Output: Album title
// Set new title for album
album.val.title = 'Updated album'
// Update album by doing a PUT request to /albums/1/
await album.update()
# Mapping of service URLs to vue-service-model methods
Url | HTTP Method | vue-service-model methods |
---|---|---|
/albums/ | GET | Album.objects.list() |
/albums/ | POST | Album.objects.create() or album.create() |
/albums/{pk}/ | GET | Album.objects.detail() or album.reload() |
/albums/{pk}/ | PUT | Album.objects.update() or album.update() |
/albums/{pk}/ | DELETE | Album.objects.delete() or album.delete() |
# Rendering:
By using a common component DisplayField
you can render the value of a field for display purpose anywhere in your application with the same output.
<display-field :model="album" field-name="title"/>
Or InputField
for an input field.
<input-field :model="album" field-name="title"/>
# Contribution
Feel free to create an issue for bugs, feature requests, suggestions or any idea you have. You can also add a pull request with your implementation.
It would please me to hear from your experience.
I used some ideas and names from django (opens new window), django REST framework (opens new window), ag-Grid (opens new window) and other libraries and frameworks.